Writing to the Bitfenix ICON display with Rust (part 1, writing images)
Jan 28 2020 · Random
Bitfenix ICON
I love the Bitfenix Pandora case and used it for my desktop PC build a few years ago. One interesting aspect about this case is that it has an attached LCD on the front, the Bitfenix ICON display; I’ve never done anything with the display (I just left the default logo on it), but recently I discovered the source code was available and it was possible to programmatically write to the display, and became interested in how this is done and what I could do with the display.
Instead of using the code provided by Bitfenix, I used the modified source produced by Rizvi R. I fired up Visual C++, dealt with downloading and pointing the compiler + linker to necessary libraries, I hit a stumbling block with libjpeg, but stripped out the JPEG support and was able to get something up and running. The code loads an image, resizes it to fit the display, reduces the color depth to match the display, and then writes to the display using HIDAPI (which is really cool and something I didn’t realize existed). The code was ok, but I decided to see if I could modularize it further and rewrite it in Rust (avoiding some of the less modern things in C++ world, like package management) as a learning exercise.
The Display
The ICON display is far from any sort of high quality panel, but it’s adequate for something put on a case. I haven’t come across official specs, but here’s what I’ve been able to gather:
- Resolution of 240×320
- 16-bit color, 5-6-5 format (5 bits for red, 6 bits for green, 5 bits for blue)
- Super slow refresh rate, like over 1 second (so not suitable for any sort of animation)
Rust Dependencies
We’ll make use of 2 Rust packages, png and hidapi via cargo:
[dependencies]
png = "0.15.3"
hidapi = "1.1.0"
Loading PNG images & converting to 16bpp
We can load a PNG image and get a pixel buffer as follows:
fn load_png_image(filepath: &str) -> Vec<u8> {
let decoder = png::Decoder::new(File::open(filepath).unwrap());
let (info, mut reader) = decoder.read_info().unwrap();
// Allocate the output buffer.
let mut buf = vec![0; info.buffer_size()];
reader.next_frame(&mut buf).unwrap();
buf
}
This will work well for 24bpp images and return a buffer with R, G, and B components, 8 bits each. As the ICON is a 5-6-5 16bpp display, we’ll need to reduce the color depth by chopping off bits with some bit shift operations (for more details on what’s happening here, this is a good resource):
fn reduce_image_to_16bit_color(image_buf: &[u8]) -> Vec<u8> {
let mut result: Vec<u8> = Vec::with_capacity(240 * 320 * 2);
for i in (0..image_buf.len()).step_by(3) {
let b: u16 = ((image_buf[i + 2] as u16) >> 3) & 0x001F;
let g: u16 = (((image_buf[i + 1] as u16) >> 2) << 5) & 0x07E0;
let r: u16 = (((image_buf[i] as u16) >> 3) << 11) & 0xF800;
let rgb: u16 = r | g | b;
result.push( ((rgb >> 8) & 0x00FF) as u8 );
result.push( (rgb & 0x00FF) as u8 );
}
result
}
We can now load an image and reduce the color depth as follows:
// Image needs to be 240x320 (24bpp, no alpha channel)
let src_image = load_png_image("assets/1.png");
let reduced_color_img = reduce_image_to_16bit_color(&src_image);
Open the ICON device
Using the HIDAPI, we can open the device with it’s vendor ID (0x1fc9) and product ID (0x100b):
let hid = HidApi::new().unwrap();
let bitfenix_icon_device = hid.open(0x1fc9, 0x100b).unwrap();
I thought it might be useful to query and surface product and manufacturer info of the device as follows:
let device_manuf_string = bitfenix_icon_device.get_manufacturer_string().unwrap().unwrap();
let device_prod_string = bitfenix_icon_device.get_product_string().unwrap().unwrap();
println!("{}: {}", device_manuf_string.as_str(), device_prod_string.as_str());
However, this isn’t terribly insightful. We get a product string equal to “NXP Semiconductors” manufacturer string equal to “LPC11Uxx HID”, which is the microcontroller used on the device.
Writing to the display
With the pixel buffer and an open device, we can now write a new image to the display, in a 3 step process:
- Clear the display
- Write the buffer to the display
- Refresh the display
I’m somewhat confused as to why the display needs to be cleared, but if I skip this step I end up with weird overdraw of pixels on top of the existing image. In any case, clearing the display is simple enough and involves writing a 6 byte code to the display:
fn clear_display(bitfenix_icon_device: &HidDevice) {
let erase_flash_code: [u8; 6] = [0x0, 0x1, 0xde, 0xad, 0xbe, 0xef];
bitfenix_icon_device.write(&erase_flash_code).unwrap();
}
Writing the buffer to the display involves copying up to 64 bytes at a time to the display, this is a max of 61 bytes from the pixel buffer + a 3 byte header (indicating the operation and number of bytes being written):
fn write_image_to_display(bitfenix_icon_device: &HidDevice, image_buf: &[u8]) {
let num_image_bytes_per_write = 61; /* +3 bytes for the header, note that the device only accepts writes in 64 bytes chunks */
let num_writes = ((image_buf.len() as f64 / num_image_bytes_per_write as f64).ceil()) as usize;
for i in 0..num_writes {
let start = i * num_image_bytes_per_write;
let mut length = num_image_bytes_per_write;
if i == (num_writes-1) {
length = image_buf.len() - ((num_writes - 1) * num_image_bytes_per_write);
}
let mut image_data_with_header: Vec<u8> = Vec::with_capacity(length + 3);
image_data_with_header.push(0x0);
image_data_with_header.push(0x2);
image_data_with_header.push(length as u8);
for image_byte_idx in start..start+length {
image_data_with_header.push(image_buf[image_byte_idx]);
}
bitfenix_icon_device.write(&image_data_with_header).unwrap();
}
}
Refreshing the display requires writing a 2 bytes code to the display:
fn refresh_display(bitfenix_icon_device: &HidDevice) {
let refresh_code: [u8; 2] = [0x0, 0x3];
bitfenix_icon_device.write(&refresh_code).unwrap();
}
Pulling it all together we have a few straightforward function calls:
println!("Writing new image...");
clear_display(&bitfenix_icon_device); // needs to be done or you end up with weird overwriting on top of exiting image
write_image_to_display(&bitfenix_icon_device, &reduced_color_img);
println!("Refreshing display...");
refresh_display(&bitfenix_icon_device);
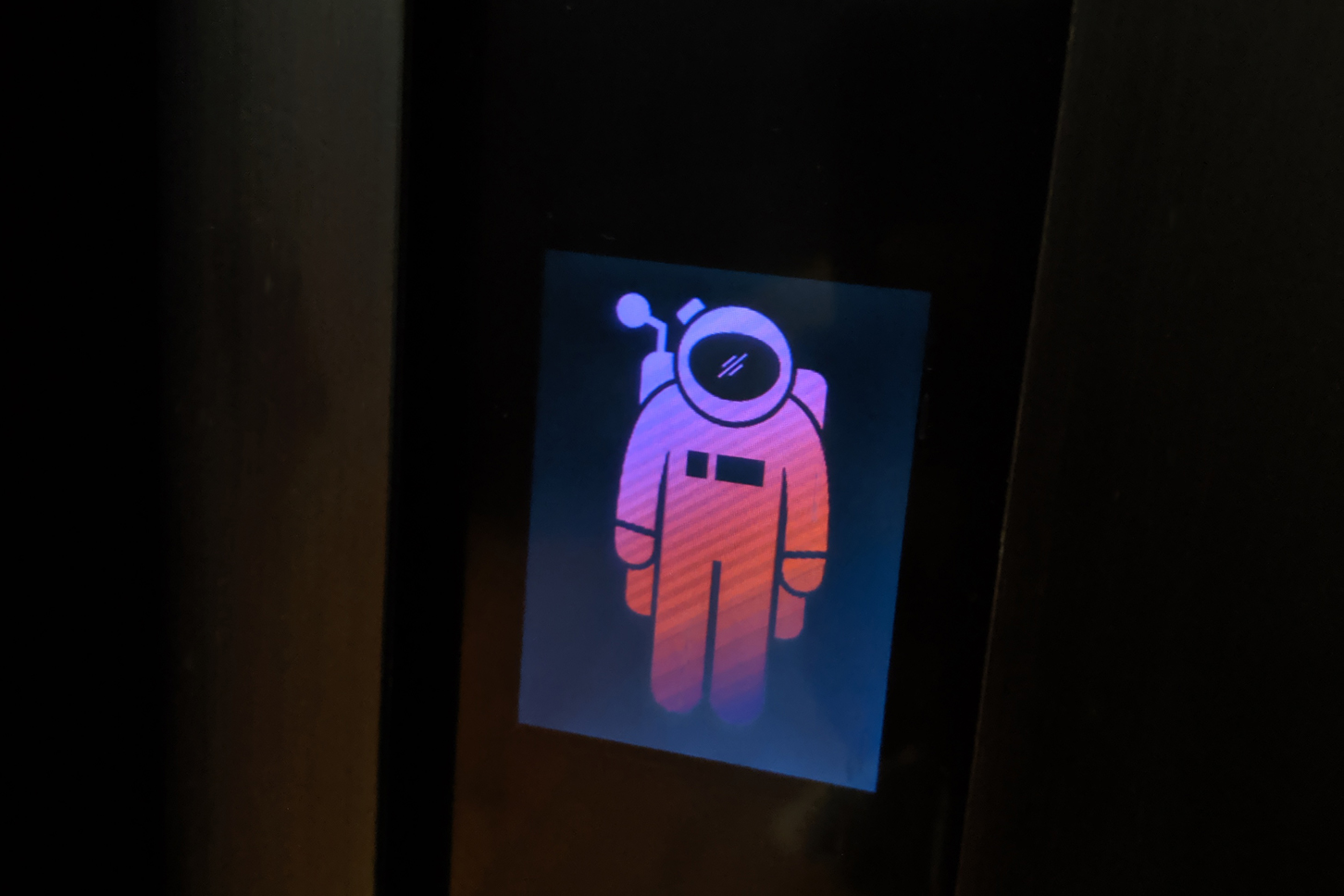
Code and future work
I have the above code up on GitHub. This was fun and a great learning experience. Next, I think it would be interesting to see if the display could be utilized to reflect information about the computer, maybe showing CPU usage or internet connection status. There could be some utility in providing such metrics to the user and it would give the display a purpose beyond that of a digital case badge.